Your cart is currently empty!
When Data Must be Sanitized, Escaped, and Validated in WordPress
In WordPress, you must sanitize, validate, and escape data at different points to ensure security and data integrity. Hereโs a simple rule to follow:
1. SANITIZE โ When Accepting Input (Before Saving to Database)
Sanitization ensures that data is clean before storing it in the database.
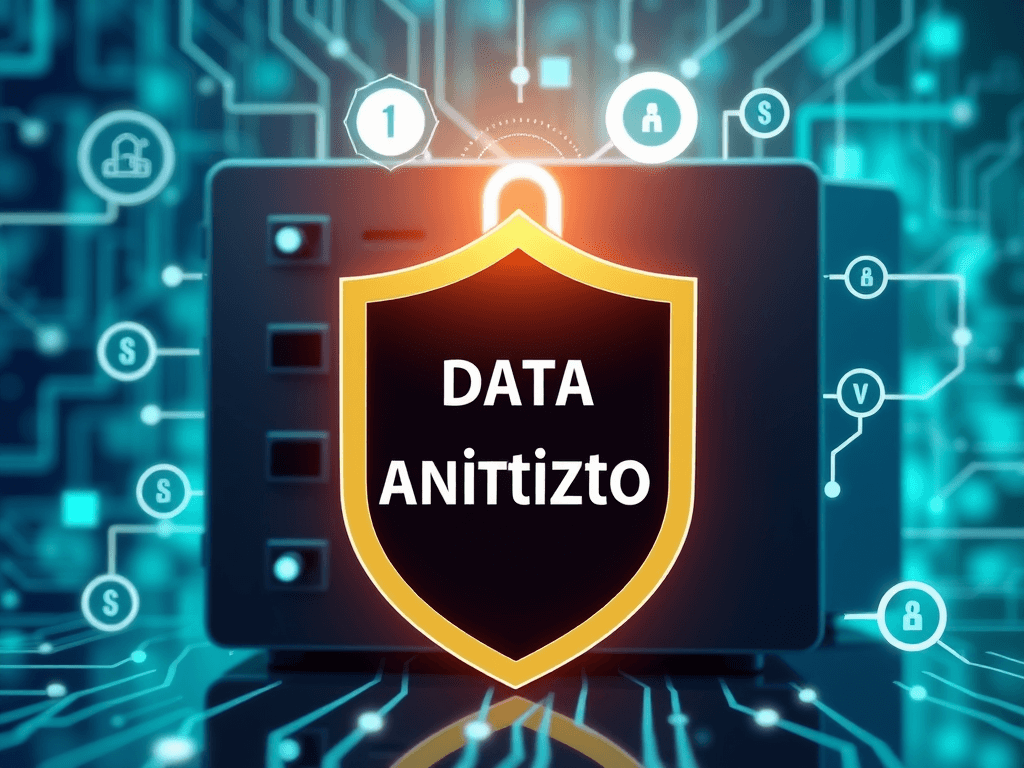
๐น When to sanitize?
- When processing
$_POST
,$_GET
,$_REQUEST
,$_FILES
, etc. - When storing data using
update_option()
,update_post_meta()
,insert_post()
, etc.
๐น How to sanitize?
Use appropriate sanitization functions based on the expected data type:
Data Type | Function to Use | Example |
---|---|---|
Text input | sanitize_text_field() | $title = sanitize_text_field( $_POST['title'] ); |
sanitize_email() | $email = sanitize_email( $_POST['email'] ); | |
URL | esc_url_raw() | $url = esc_url_raw( $_POST['url'] ); |
Integer | absint() | $age = absint( $_POST['age'] ); |
Float | floatval() | $price = floatval( $_POST['price'] ); |
HTML | wp_kses_post() (allowed HTML) | $content = wp_kses_post( $_POST['content'] ); |
Checkbox (Boolean) | boolval() | $checked = boolval( $_POST['checkbox'] ); |
JSON | json_decode() + array_map() | $data = json_decode( stripslashes( $_POST['json_data'] ), true ); array_walk_recursive( $data, 'sanitize_text_field' ); |
โ Example: Correct Way to Sanitize Data Before Saving
if ( isset( $_POST['icwcu_custom_services_title'] ) ) {
$custom_services_title = sanitize_text_field( wp_unslash( $_POST['icwcu_custom_services_title'] ) );
update_post_meta( $post_id, 'icwcu_custom_services_title', $custom_services_title );
}
2. VALIDATE โ Before Using the Data
Validation ensures that the sanitized data is in the expected format.
๐น When to validate?
- When checking if data meets specific criteria (e.g., is an email valid? Is a number in a certain range?)
- Before processing critical information (e.g., order amounts, email addresses, etc.).
๐น How to validate?
Use proper WordPress functions or custom validation logic:
Data Type | Validation Function | Example |
---|---|---|
is_email() | if ( ! is_email( $email ) ) { return new WP_Error( 'invalid_email', 'Invalid email address' ); } | |
Integer | is_numeric() | if ( ! is_numeric( $price ) ) { return new WP_Error( 'invalid_number', 'Price must be a number' ); } |
URL | filter_var( $url, FILTER_VALIDATE_URL ) | if ( ! filter_var( $url, FILTER_VALIDATE_URL ) ) { return new WP_Error( 'invalid_url', 'Invalid URL' ); } |
Boolean | in_array( $value, [ 'yes', 'no' ] ) | if ( ! in_array( $value, [ 'yes', 'no' ] ) ) { return new WP_Error( 'invalid_choice', 'Invalid value' ); } |
โ Example: Validating Data Before Using
$email = sanitize_email( $_POST['email'] );
if ( ! is_email( $email ) ) {
wp_die( 'Invalid email address provided.' );
}
3. ESCAPE โ When Outputting Data
Escaping ensures that data is safe when displayed on the screen, preventing XSS (Cross-Site Scripting) attacks.
๐น When to escape?
- When displaying data in HTML, JavaScript, URLs, or attributes.
- When using
echo
,printf()
,return
, etc.
๐น How to escape?
Use esc_*() functions based on where the data is being output:
Context | Function to Use | Example |
---|---|---|
HTML Text | esc_html() | echo esc_html( $name ); |
HTML Attributes | esc_attr() | <input type="text" value="<?php echo esc_attr( $name ); ?>"> |
URLs | esc_url() | <a href="<?php echo esc_url( $url ); ?>">Link</a> |
JavaScript | esc_js() | <script>var name = "<?php echo esc_js( $name ); ?>";</script> |
SQL Queries | esc_sql() | $wpdb->prepare( "SELECT * FROM table WHERE column = %s", esc_sql( $value ) ); |
โ Example: Escaping Data Before Displaying
echo '<h2>' . esc_html( get_option( 'icwcu_custom_services_title' ) ) . '</h2>';
echo '<a href="' . esc_url( get_option( 'icwcu_custom_services_link' ) ) . '">Click Here</a>';
Final Cheat Sheet
Action | When to Use | Example Function |
---|---|---|
Sanitize | Before saving to database | sanitize_text_field() , sanitize_email() , esc_url_raw() , absint() |
Validate | Before processing user input | is_email() , is_numeric() , filter_var( $url, FILTER_VALIDATE_URL ) |
Escape | Before outputting to screen | esc_html() , esc_attr() , esc_url() , esc_js() |
Conclusion
- Sanitize input before storing it ๐ (e.g., from
$_POST
,$_GET
,$_REQUEST
). - Validate input before processing it โ (e.g., checking formats).
- Escape output before displaying it ๐ (e.g., when using
echo
,print
, HTML).
This will help you to make a secure WordPress plugin or theme! ๐
Comments
Grabber Pro
Original price was: $59.$39Current price is: $39.Custom WooCommerce Checkbox Ultimate
Original price was: $39.$19Current price is: $19.Android App for Your Website
Original price was: $49.$35Current price is: $35.Abnomize Pro
Original price was: $30.$24Current price is: $24.Medical Portfolio Pro
Original price was: $31.$24Current price is: $24.
Latest Posts
- How to Create a PHP Remote File Downloader with Live Progress Bar
- How to Connect AWS CloudFront URL with a Cloudflare Subdomain
- Android Developer Interview Questions Categorized by Topic
- When Data Must be Sanitized, Escaped, and Validated in WordPress
- Alternative to WordPress for High Traffic News Websites: Node.js & Other Scalable Solutions
Leave a Reply