Your cart is currently empty!
In many Android applications, the ability to upload user-selected files, such as images or PDF documents, to a server is essential. This tutorial will guide you step-by-step through the process of implementing image and PDF file uploads in your Android app. We’ll leverage the popular Retrofit library to simplify the interaction with your server-side API.
Prerequisites
- Basic understanding of Android development with Java or Kotlin.
- A working Android Studio project.
- A server-side API endpoint that can receive and process uploaded files.
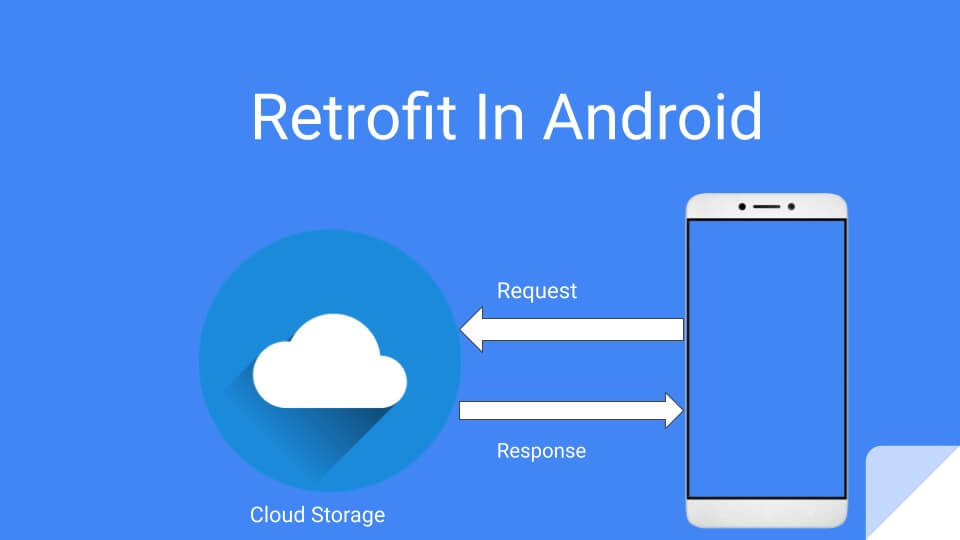
Step 1: Project Setup
- Dependencies: Add the following dependencies to your project’s
build.gradle
file:
dependencies {
implementation("com.squareup.retrofit2:retrofit:2.9.0") // Update to latest if needed
implementation("com.squareup.retrofit2:converter-gson:2.9.0")
implementation("com.squareup.okhttp3:okhttp:4.10.0") // Update to latest if needed
}
Permissions: Ensure your app has storage access. Add the following line to your AndroidManifest.xml
:
<uses-permission android:name="android.permission.READ_EXTERNAL_STORAGE" />
Step 2: Android Code
Here’s an improved version of your UploadPodActivity
(adjusting package names and API details as needed):
import android.Manifest;
import android.content.Intent;
import android.content.pm.PackageManager;
import android.database.Cursor;
import android.net.Uri;
import android.os.Bundle;
import android.provider.OpenableColumns;
import android.util.Log;
import android.view.View;
import android.widget.Toast;
import androidx.activity.result.ActivityResultLauncher;
import androidx.activity.result.contract.ActivityResultContracts;
import androidx.appcompat.app.AppCompatActivity;
import androidx.core.app.ActivityCompat;
import androidx.core.content.ContextCompat;
import java.io.File;
import java.io.FileOutputStream;
import java.io.InputStream;
import okhttp3.MediaType;
import okhttp3.MultipartBody;
import okhttp3.RequestBody;
import retrofit2.Call;
import retrofit2.Callback;
import retrofit2.Response;
import retrofit2.Retrofit;
import retrofit2.converter.gson.GsonConverterFactory;
public class UploadPodActivity extends AppCompatActivity {
// ... (Other UI elements and SharedPreferences code)
private static final int REQUEST_READ_STORAGE = 100;
@Override
protected void onCreate(Bundle savedInstanceState) {
// ... (Existing onCreate code)
// ... (OnClickListener setup)
}
private void chooseFile() {
if (ContextCompat.checkSelfPermission(this, Manifest.permission.READ_EXTERNAL_STORAGE)
!= PackageManager.PERMISSION_GRANTED) {
ActivityCompat.requestPermissions(this,
new String[]{Manifest.permission.READ_EXTERNAL_STORAGE},
REQUEST_READ_STORAGE);
} else {
openFilePicker();
}
}
private void openFilePicker() {
Intent intent = new Intent(Intent.ACTION_GET_CONTENT);
intent.setType("*/*");
String[] mimeTypes = {"image/*", "application/pdf"};
intent.putExtra(Intent.EXTRA_MIME_TYPES, mimeTypes);
resultLauncher.launch(intent);
}
private ActivityResultLauncher<Intent> resultLauncher = registerForActivityResult(
new ActivityResultContracts.StartActivityForResult(),
result -> {
// ... (Handle file selection in onActivityResult)
});
// ... (Other utility methods: getFileFromContentUri, getFileNameFromUri)
// ... (uploadFile method with Retrofit API setup)
}
Step 3: Create Your Retrofit Interface
Define an interface for your API interaction:
// File: ApiService.java
import retrofit2.Call;
import retrofit2.http.Multipart;
import retrofit2.http.POST;
import retrofit2.http.Part;
import retrofit2.http.Header;
interface ApiService {
@Multipart
@POST("your_upload_endpoint") // Replace with your API endpoint
Call<PodUploadResponse> uploadPOD(@Header("Authorization") String authorization,
@Part MultipartBody.Part file,
@Part("docketNo") RequestBody docketNo,
You can Download Full Code Here
Comments
Grabber Pro
Original price was: $59.$39Current price is: $39.Insertcart Custom WooCommerce Checkbox Ultimate
Original price was: $39.$19Current price is: $19.Android App for Your Website
Original price was: $49.$35Current price is: $35.Abnomize Pro
Original price was: $30.$24Current price is: $24.Medical Portfolio Pro
Original price was: $31.$24Current price is: $24.
Latest Posts
- Build a Simple PHP Note-Taking App with AJAX
- How to Dynamically Add or Remove Classes Based on Screen Size Using jQuery
- How to Handle Sudden Traffic Spike in Website – Do Node Balancer Really Help
- How to Use AWS SES Email from Localhost or Website: Complete Configuration in PHP
- How to Upload Images and PDFs in Android Apps Using Retrofit
Leave a Reply