Your cart is currently empty!
Get answers to your most pressing Android development questions. We cover everything from the basics of Activities and Intents to complex topics like background restrictions, app bundles, and efficient data management. Level up your Android skills today.
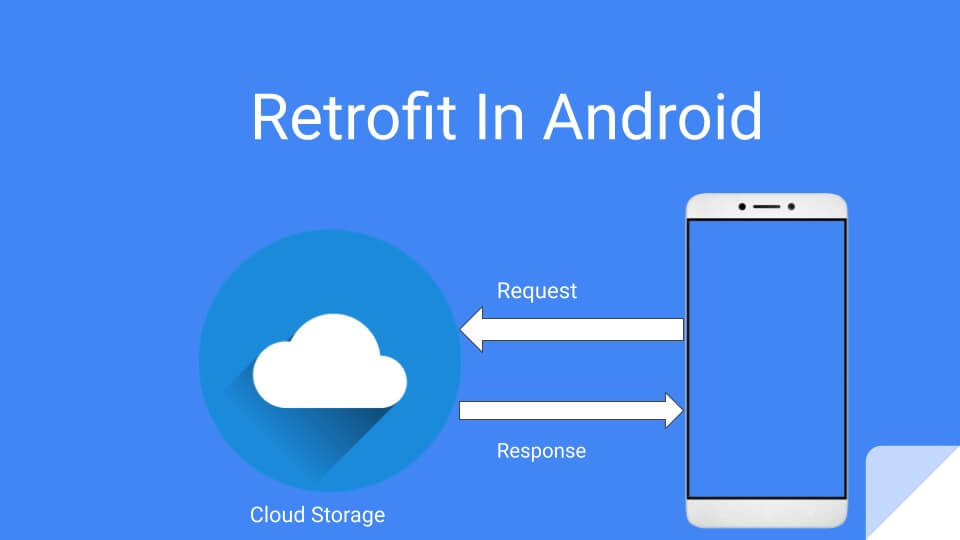
Core Android Development
- What are the main components of an Android application?
- Android applications consist of four main components:
- Activities: Represent a single, focused thing that the user can do. They provide the user interface.
- Services: Run in the background to perform long-running operations or to perform work for remote processes.
- Broadcast Receivers: Respond to system-wide broadcast announcements.
- Content Providers: Manage a shared set of application data that you can store in the file system, in an SQLite database, or in any other persistent storage location your application can access.
- Android applications consist of four main components:
- What is the difference between an Activity and a Fragment?
- Activity:
- Represents a single, independent screen with a user interface.
- Has its own lifecycle.
- Can exist independently.
- Fragment:
- Represents a portion of a user interface in an Activity.
- Has its own lifecycle, but it’s dependent on the Activity’s lifecycle.
- Must be hosted within an Activity.
- Fragments are designed to be reusable and flexible, especially for adapting to different screen sizes.
- Activity:
- How does the Android lifecycle work? Explain each state of an Activity.
- The Android Activity lifecycle defines the states an Activity can go through during its lifetime. The main states are:
- onCreate(): Called when the Activity is first created. Initialization occurs here.
- onStart(): Called when the Activity becomes visible to the user.
- onResume(): Called when the Activity starts interacting with the user. It’s in the foreground.
- onPause(): Called when the system is about to resume another Activity. It’s partially visible, but not interacting.
- onStop(): Called when the Activity is no longer visible to the user.
- onRestart(): Called after the Activity has been stopped, just prior to it being started again.
- onDestroy(): Called before the Activity is destroyed.2 This is the final call.
- The Android Activity lifecycle defines the states an Activity can go through during its lifetime. The main states are:
- What is a Service in Android? How is it different from an IntentService?
- Service:
- A background process that can perform long-running operations without a user interface.
- Runs on the main thread by default.
- Requires manual thread management for long-running tasks.
- IntentService:
- A subclass of Service that handles asynchronous requests (intents) on a single background thread.
- Automatically stops itself when it’s done processing all intents.
- Is now depreciated, and WorkManager is now the prefered method.
- The main difference is that IntentService handles tasks sequentially on a separate thread, simplifying background processing, while a regular Service requires you to manage threading yourself.
- Service:
- What are Broadcast Receivers, and when would you use them?
- Broadcast Receivers are components that respond to system-wide broadcast announcements.
- They can be used to:
- React to system events (e.g., low battery, network connectivity changes).
- Receive broadcasts from other applications.
- Trigger actions based on specific events.
- What is the difference between implicit and explicit intents?
- Explicit Intent:
- Specifies the exact component (Activity, Service, etc.) that should handle the intent.
- Uses the component’s class name.
- Used when you know precisely which component you want to start.
- Implicit Intent:
- Declares the action to be performed (e.g., ACTION_VIEW, ACTION_SEND).
- The system determines which component can handle the intent based on intent filters.
- Used when you want to perform an action but don’t know which specific component should handle it.
- Explicit Intent:
- What are Content Providers, and why are they useful?
- Content Providers manage access to a structured set of data.
- They are useful for:
- Sharing data between applications.
- Providing a standardized interface for accessing data.
- Encapsulating data storage and retrieval.
UI & UX
- What is a RecyclerView, and how is it different from a ListView?
- RecyclerView:
- A more advanced and flexible view for displaying a scrolling list of elements.
- Uses a ViewHolder pattern for efficient view recycling.
- Has a pluggable layout manager for different list layouts.
- Offers better performance for large datasets.
- ListView:
- A simpler view for displaying a scrolling list.
- Less efficient for large datasets.
- Less flexible in terms of layout management.
- RecyclerView is the modern and preferred approach for displaying lists.
- RecyclerView:
- How do you handle configuration changes (e.g., screen rotation) in Android?
- Configuration changes cause the Activity to be destroyed and recreated by default.
- To handle them:
- ViewModel: Use ViewModel to store UI state that survives configuration changes.
- onSaveInstanceState() / onRestoreInstanceState(): Save and restore transient UI state.
- android:configChanges: (Use with caution) Declare configuration changes in the manifest to handle them yourself.
- What is ViewModel, and how does it help in UI state management?
- ViewModel:
- A class designed to store and manage UI-related data in a lifecycle-conscious way.
- Survives configuration changes.
- Separates UI data from the UI controller (Activity/Fragment).
- Helps to prevent memory leaks and improve testability.
- ViewModel:
- What are Jetpack Compose and XML-based UI? When should you use each?
- Jetpack Compose:
- A modern, declarative UI toolkit.
- Uses Kotlin code to define UI.
- Offers a more concise and reactive approach.
- Best for new projects, or projects that are wanting to modernize their UI.
- XML-based UI:
- The traditional Android UI toolkit.
- Uses XML files to define layouts.
- Mature and widely supported.
- Best for maintaining older projects, or projects that require a very stable and well understood UI system.
- Compose is the future, but XML is still widely used.
- Jetpack Compose:
- What is Data Binding, and how does it differ from View Binding?
- View Binding:
- Generates binding classes that allow you to access views in XML layouts directly in code.
- Provides compile-time null safety.
- Reduces boilerplate code for finding views.
- Data Binding:
- Extends View Binding by allowing you to bind data directly to views in XML layouts.
- Supports two-way data binding.
- Reduces boilerplate code for updating UI based on data changes.
- Data binding is more powerful, as it can bind variables directly to the layout, and view binding is only for referencing the views.
- View Binding:
Data Storage & Management
- What is Room Database, and how does it improve SQLite?
- Room is a persistence library that provides an abstraction layer over SQLite.
- It improves SQLite by:
- Providing compile-time verification of SQL queries.2
- Reducing boilerplate code.3
- Integrating seamlessly with LiveData and RxJava.
- Handling thread safety automatically.
- What is SharedPreferences, and when should it be used?
- SharedPreferences is used to store small amounts of key-value data.4
- It should be used for:
- Storing user preferences.
- Saving simple application settings.
- Persisting small, non-critical data.
- How can you store large amounts of structured data efficiently in an Android app?
- For large amounts of structured data:
- Use Room Database for relational data.
- Use Protocol Buffers or JSON for structured data in files.
- Consider using a NoSQL database (like Firebase Realtime Database or Firestore) for cloud-based storage.7
- For large amounts of structured data:
- What are WorkManager and AlarmManager, and how do they differ?
- WorkManager:
- Used for deferrable background work that needs to be guaranteed to execute.
- Takes into account device constraints (battery, network).
- Handles background work even if the app is closed.
- AlarmManager:
- Used to schedule tasks to run at a specific time or interval.
- Less reliable than WorkManager for guaranteed execution.
- Suitable for time-based events.
- WorkManager is for deferrable background work, and AlarmManager is for time based events.
- WorkManager:
Networking & APIs
- What is Retrofit, and how does it compare to Volley?
- Retrofit:
- A type-safe REST client for Android and Java.
- Uses annotations to define API endpoints.
- Integrates well with OkHttp.
- Volley:
- A networking library by Google.
- Handles network operations asynchronously.
- Provides caching and request prioritization.
- Retrofit is generally preferred for its ease of use and type safety, while Volley is a more general-purpose networking library.
- Retrofit:
- How do you handle API responses and errors in Android?
- Use try-catch blocks to handle exceptions.
- Check HTTP status codes for success or failure.
- Use data classes to model API responses.
- Implement error handling in a centralized way.
- What is OkHttp, and how does it enhance networking in Android?
- OkHttp:
- An efficient HTTP client for Android and Java.
- Supports HTTP/2, connection pooling, and GZIP compression.
- Enhances networking by:
- Improving performance.
- Reducing latency.
- Simplifying network requests.
- OkHttp:
- How would you implement WebSockets in an Android app?
- Use OkHttp’s WebSocket API.
- Establish a WebSocket connection to the server.
- Send and receive messages using the WebSocket interface.
- Libraries like Socket.IO also simplify Websocket implementation.
Security & Performance
- How do you secure sensitive data in an Android app?
- Use encryption (e.g., AES) for sensitive data.
- Store keys in the Android Keystore.
- Avoid hardcoding sensitive data in the app.
- Use HTTPS for network communication.
- Use code obfuscation.
- What are ProGuard and R8, and how do they help in Android development?
- ProGuard/R8:
- Tools for code shrinking, obfuscation, and optimization.
- Help in Android development by:
- Reducing app size.
- Making reverse engineering more difficult.
- Improving performance.
- R8 is the newer and more efficient code shrinker and optimizer.
- ProGuard/R8:
- What are memory leaks in Android, and how do you prevent them?
- Memory leaks occur when objects are no longer needed but are still referenced.
- Prevent them by:
- Unregistering listeners.
- Avoiding static references to Activities.
- Using WeakReference to prevent strong references.
- Using Android Studio’s memory profiler.
- What is ANR (Application Not Responding), and how do you avoid it?
- ANR occurs when the UI thread is blocked for too long.
- Avoid it by:
- Performing long-running operations on background threads.
- Using AsyncTask, Coroutines, or RxJava for background tasks.
- Optimizing UI performance.
- How can you optimize an Android app for better performance?
- Optimize layouts.
- Use efficient data structures.
- Minimize network requests.
- Use image compression.
- Profile app performance with Android Studio tools.
Multithreading & Concurrency
- What is the difference between AsyncTask, Coroutines, and RxJava?
- AsyncTask:
- Deprecated.
- Simplified background task execution.
- Prone to memory leaks.
- Coroutines:
- Lightweight threads for asynchronous programming in Kotlin.
- Simplified asynchronous code with suspend functions.
- Preferred for modern Android development.
- RxJava:
- Reactive programming library for asynchronous and event-based programs.
- Powerful for complex asynchronous operations.
- Can have a steeper learning curve.
- AsyncTask:
- How does the Handler class work in Android?
- Handler allows you to post and execute tasks on a specific thread’s message queue.
- Used for updating the UI thread from background threads.
- What is LiveData, and how is it different from MutableLiveData?
- LiveData:
- A lifecycle-aware observable data holder.
- Updates observers when data changes.
- Ensures UI updates only when the observer is active.
- MutableLiveData:
- A subclass of LiveData that allows you to modify the data.
- Used to update LiveData values.
- LiveData:
- How does Kotlin Flow improve handling of async data streams?
- Kotlin Flow provides a way to handle streams of data asynchronously.
- It is built on coroutines, and enables backpressure.
- It is very effective for handling sequences of data over time, such as network responses, or database changes.
- What are Executors, and when would you use them?
- Executors are used to manage threads.
- You would use them when you need to run background tasks that are not UI related.
- They are good for running code on thread pools.
Jetpack Components & Modern Android Development
- What is MVVM, and how is it different from MVP?
- MVVM (Model-View-ViewModel):
- Separates UI logic from business logic using a ViewModel.
- Uses data binding to update the UI.
- ViewModel is lifecycle aware.
- MVP (Model-View-Presenter):
- Separates UI logic from business logic using a Presenter.
- Presenter handles UI updates.
- View is passive.
- MVVM is more modern, and uses data binding to reduce code.
- MVVM (Model-View-ViewModel):
- What is Hilt, and how does it simplify dependency injection?
- Hilt is a dependency injection library for Android.
- It simplifies dependency injection by:
- Generating boilerplate code.
- Providing standard ways to inject dependencies.
- Integrating with Jetpack components.
- Explain Paging 3 and how it helps with loading large datasets efficiently.
- Paging 3 is a library that helps load large datasets in chunks.41
- It helps by:
- Loading data on demand.
- Reducing memory usage.
- Providing smooth scrolling.
- What is Navigation Component, and how does it improve app navigation?
- Navigation Component is a library for handling in-app navigation.42
- It improves navigation by:
- Providing a visual navigation graph.
- Simplifying fragment transactions.
- Handling deep linking.
Android System & Advanced Topics
- What is the difference between implicit and explicit broadcast receivers?
- Explicit Broadcast Receiver:
- Specifically targets a particular application component (receiver) by its fully qualified class name.
- Only the targeted component will receive the broadcast.
- More secure, as you know exactly who will receive the broadcast.
- Implicit Broadcast Receiver:
- Declares an action that it can handle, and the system determines which components are registered to receive that action.
- Any app that has registered an intent filter for the specified action can receive the broadcast.
- Can pose security risks if not used carefully, as unintended apps might receive the broadcast.
- Explicit Broadcast Receiver:
- What is Scoped Storage, and how has it changed file access in Android?
- Scoped Storage:
- A set of Android platform changes that restrict how applications can access files on external storage.
- It aims to improve user privacy and security by limiting the scope of file access.
- Changes:
- Apps are primarily limited to accessing their own app-specific directories and media files that they have created.
- Access to shared media collections (images, videos, etc.) is mediated through system pickers and content URIs.
- Raw file system paths are less accessible.
- Essentially, it has increased the level of sandboxing that each application has when accessing the devices file system.
- Scoped Storage:
- What are Background Restrictions, and how do they impact app behavior?
- Background Restrictions:
- Android’s mechanisms for limiting the ability of apps to run in the background.
- These restrictions are designed to conserve battery life and system resources.
- Impacts:
- Background services might be stopped.
- Background network access might be limited.
- Background location updates might be less frequent.
- WorkManager is designed to work within these limitations.
- Android has become more and more aggressive about limiting background activity.
- Background Restrictions:
- How does Android handle multiple processes in an application?
- Android applications can run in one or more processes.
- By default, all components of an app run in the same process.
- Apps can create additional processes by specifying the
android:process
attribute in their manifest. - Inter-process communication (IPC) is used to allow processes to communicate with each other.
- This can be done using:
- Intents.
- Content Providers.
- AIDL (Android Interface Definition Language).
- Bound services.
- This can be done using:
- Android’s process management system dynamically allocates and reclaims resources based on app usage and system conditions.
- What is Android App Bundles, and how do they improve app distribution?
- Android App Bundles:
- A publishing format that includes all of your app’s compiled code and resources, but defers APK generation and signing to Google Play.
- Google Play’s Dynamic Delivery then uses your app bundle to generate and serve optimized APKs for each user’s device configuration.
- Improvements:
- Smaller App Sizes: Users download only the code and resources they need.
- Dynamic Feature Delivery: Allows for on-demand delivery of app features.
- Asset Delivery: Allows for the delivery of high quality game assets, on demand.
- Improved Distribution: More efficient distribution and storage of application files.
- Android App Bundles:
Learn the key concepts and techniques for building high-quality Android applications. This article covers essential topics like data storage, networking, security, and advanced system features, providing practical insights for developers of all levels.
Comments
Grabber Pro
Original price was: $59.$39Current price is: $39.Custom WooCommerce Checkbox Ultimate
Original price was: $39.$19Current price is: $19.Android App for Your Website
Original price was: $49.$35Current price is: $35.Abnomize Pro
Original price was: $30.$24Current price is: $24.Medical Portfolio Pro
Original price was: $31.$24Current price is: $24.
Latest Posts
- How to Create a PHP Remote File Downloader with Live Progress Bar
- How to Connect AWS CloudFront URL with a Cloudflare Subdomain
- Android Developer Interview Questions Categorized by Topic
- When Data Must be Sanitized, Escaped, and Validated in WordPress
- Alternative to WordPress for High Traffic News Websites: Node.js & Other Scalable Solutions
Leave a Reply