Your cart is currently empty!
If you’re looking to create a lightweight and efficient note-taking application, this tutorial will guide you through the process of building one using PHP, MySQL, AJAX, and a rich text editor like TinyMCE. This guide is beginner-friendly and SEO-optimized for keywords like “PHP note-taking app,” “AJAX CRUD operations,” and “MySQL database integration.”
Why Build a Note-Taking App?
A note-taking app can be a handy tool for organizing thoughts, tasks, or project details. By building it yourself, you gain valuable experience in web development while customizing the app to your exact needs. With features like real-time saving, editing, and deleting notes, this app ensures a seamless user experience.
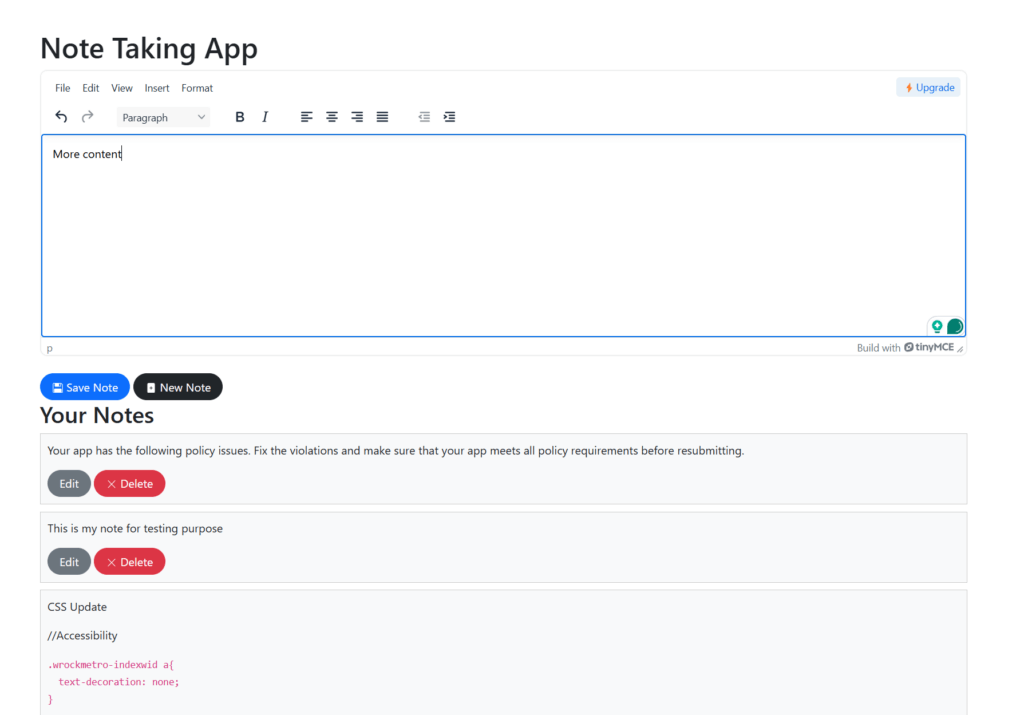
Features of the Note-Taking App
- Rich Text Editor: Integrates TinyMCE for formatting text.
- AJAX Operations: Handles create, read, update, and delete (CRUD) actions without refreshing the page.
- Database Integration: Stores notes in a MySQL database for persistence.
- User-Friendly Interface: Simple design with intuitive buttons for adding, editing, and deleting notes.
Implementation Steps
1. Prerequisites
Before you start, ensure you have:
- A local or remote server with PHP and MySQL support (e.g., XAMPP, WAMP, or a hosting provider).
- Basic knowledge of PHP, HTML, and JavaScript.
2. Set Up the MySQL Database
Create a database named note_taking_app
and run the following SQL to set up the notes
table:
CREATE TABLE IF NOT EXISTS notes (
id INT AUTO_INCREMENT PRIMARY KEY,
content TEXT NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
);
3. The PHP Script
Hereโs the core PHP script to handle database connections and AJAX requests:
<?php
$host = 'localhost';
$user = 'root';
$password = '';
$dbname = 'note_taking_app';
$conn = new mysqli($host, $user, $password, $dbname);
if ($conn->connect_error) {
die("Connection failed: " . $conn->connect_error);
}
$tableCheckQuery = "
CREATE TABLE IF NOT EXISTS notes (
id INT AUTO_INCREMENT PRIMARY KEY,
content TEXT NOT NULL,
created_at TIMESTAMP DEFAULT CURRENT_TIMESTAMP
)
";
$conn->query($tableCheckQuery);
if ($_SERVER['REQUEST_METHOD'] === 'POST') {
$action = $_POST['action'];
$noteId = $_POST['note_id'] ?? null;
$content = $_POST['content'] ?? '';
if ($action === 'save') {
if ($noteId) {
$stmt = $conn->prepare("UPDATE notes SET content = ? WHERE id = ?");
$stmt->bind_param('si', $content, $noteId);
} else {
$stmt = $conn->prepare("INSERT INTO notes (content) VALUES (?)");
$stmt->bind_param('s', $content);
}
$stmt->execute();
echo $noteId ?: $conn->insert_id;
exit;
}
if ($action === 'fetch') {
$result = $conn->query("SELECT * FROM notes ORDER BY id DESC");
$notes = [];
while ($row = $result->fetch_assoc()) {
$notes[] = $row;
}
echo json_encode($notes);
exit;
}
if ($action === 'delete') {
if ($noteId) {
$stmt = $conn->prepare("DELETE FROM notes WHERE id = ?");
$stmt->bind_param('i', $noteId);
$stmt->execute();
echo "success";
} else {
echo "error";
}
exit;
}
}
?>
4. Frontend: HTML and JavaScript
The frontend consists of a rich text editor, buttons for saving and creating notes, and a container for displaying notes. Hereโs the code:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Note Taking App</title>
<script src="https://cdn.tiny.cloud/1/no-api-key/tinymce/6/tinymce.min.js" referrerpolicy="origin"></script>
<link href="https://cdn.jsdelivr.net/npm/[email protected]/dist/css/bootstrap.min.css" rel="stylesheet">
<script>
tinymce.init({ selector: '#editor' });
document.addEventListener('DOMContentLoaded', function () {
fetchNotes();
document.getElementById('save-note').addEventListener('click', function () {
const noteId = document.getElementById('note-id').value;
const content = tinymce.get('editor').getContent();
saveNote(noteId, content);
});
document.getElementById('new-note').addEventListener('click', function () {
document.getElementById('note-id').value = '';
tinymce.get('editor').setContent('');
});
});
function fetchNotes() {
fetch('index.php', {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: new URLSearchParams({ action: 'fetch' })
})
.then(response => response.json())
.then(notes => {
const container = document.getElementById('notes-container');
container.innerHTML = '';
notes.forEach(note => {
const div = document.createElement('div');
div.classList.add('note');
div.innerHTML = `
<div class="note-content">${note.content}</div>
<button onclick="editNote(${note.id}, \`${note.content}\`)">Edit</button>
<button onclick="deleteNote(${note.id})">Delete</button>
`;
container.appendChild(div);
});
});
}
function saveNote(noteId, content) {
fetch('index.php', {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: new URLSearchParams({ action: 'save', note_id: noteId, content })
})
.then(() => {
fetchNotes();
document.getElementById('new-note').click();
});
}
function deleteNote(noteId) {
if (confirm('Are you sure you want to delete this note?')) {
fetch('index.php', {
method: 'POST',
headers: { 'Content-Type': 'application/x-www-form-urlencoded' },
body: new URLSearchParams({ action: 'delete', note_id: noteId })
})
.then(() => fetchNotes());
}
}
function editNote(id, content) {
document.getElementById('note-id').value = id;
tinymce.get('editor').setContent(content);
}
</script>
</head>
<body>
<h1>Note Taking App</h1>
<textarea id="editor"></textarea>
<input type="hidden" id="note-id">
<button id="save-note">Save Note</button>
<button id="new-note">New Note</button>
<h2>Your Notes</h2>
<div id="notes-container"></div>
</body>
</html>
5. Test Your App
- Run the PHP script on your server.
- Add, edit, and delete notes to ensure everything works seamlessly.
Download Full Source Code with Offline files
Conclusion
This PHP note-taking app demonstrates the power of combining PHP, MySQL, and AJAX for dynamic web applications. With the addition of TinyMCE, users can format their notes, making the app versatile and user-friendly.
Comments
Grabber Pro
Original price was: $59.$39Current price is: $39.Insertcart Custom WooCommerce Checkbox Ultimate
Original price was: $39.$19Current price is: $19.Android App for Your Website
Original price was: $49.$35Current price is: $35.Abnomize Pro
Original price was: $30.$24Current price is: $24.Medical Portfolio Pro
Original price was: $31.$24Current price is: $24.
Latest Posts
- Build a Simple PHP Note-Taking App with AJAX
- How to Dynamically Add or Remove Classes Based on Screen Size Using jQuery
- How to Handle Sudden Traffic Spike in Website – Do Node Balancer Really Help
- How to Use AWS SES Email from Localhost or Website: Complete Configuration in PHP
- How to Upload Images and PDFs in Android Apps Using Retrofit
Leave a Reply