Your cart is currently empty!
How to Dynamically Add or Remove Classes Based on Screen Size Using jQuery
When creating responsive web designs, you may need to adjust styles dynamically based on the screen size. While CSS media queries are great, there are times when JavaScript provides better control. In this tutorial, you’ll learn how to use jQuery to add or remove classes from an element depending on the window size.
What You’ll Learn:
- How to dynamically check the screen width using jQuery.
- Adding and removing classes based on the screen size.
- Ensuring your code runs efficiently on page load and during window resize events.
Prerequisites:
Before starting, ensure you have:
- A basic understanding of HTML, CSS, and JavaScript.
- The jQuery library included in your project.
Step-by-Step Implementation
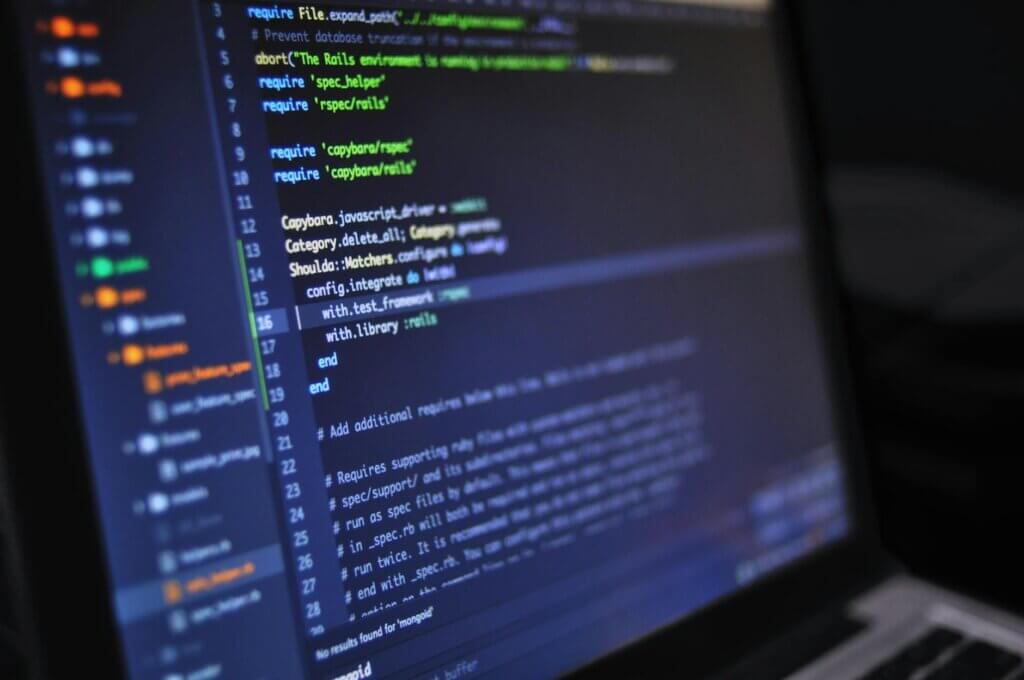
Step 1: Include jQuery in Your Project
First, include the jQuery library in your HTML file. You can use a CDN for quick setup:
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
Step 2: Write the HTML
Create an element with a class name that will change dynamically based on the screen size. For example:
<div class="mobilescr">This is a responsive element.</div>
Step 3: Add the JavaScript
Hereโs the full JavaScript code to dynamically add or remove classes based on the screen width:
function resize() {
if ($(window).width() < 700) {
$('.mobilescr').addClass('align-items-center justify-content-center');
} else {
$('.mobilescr').removeClass('align-items-center justify-content-center');
}
}
// Run the function on page load
$(document).ready(function() {
resize();
});
// Watch for window resize events
$(window).on('resize', function() {
resize();
});
How It Works:
- The
resize
Function:- Checks the current window width using
$(window).width()
. - If the width is less than 700px, it adds the
align-items-center
andjustify-content-center
classes to the.mobilescr
element. - If the width is 700px or more, it removes those classes.
- Checks the current window width using
- Run on Page Load:
- When the page loads, the
resize
function is called to ensure the correct classes are applied immediately.
- When the page loads, the
- Run on Window Resize:
- The
resize
function is called every time the window is resized to dynamically adjust the classes.
- The
Step 4: Style the Classes
Make sure the classes youโre adding are defined in your CSS. If youโre using Bootstrap, these classes are already predefined. For custom styles, you can add something like this:
.align-items-center {
align-items: center;
display: flex;
}
.justify-content-center {
justify-content: center;
}
Step 5: Optimize for Performance (Optional)
For better performance during frequent resize events, debounce the resize
function to prevent it from firing too many times:
let resizeTimeout;
$(window).on('resize', function() {
clearTimeout(resizeTimeout);
resizeTimeout = setTimeout(resize, 100); // Adjust the timeout as needed
});
Features of This Approach:
- Dynamic Control: Adds and removes classes based on the window width.
- Responsive Design: Works seamlessly with CSS frameworks like Bootstrap.
- Efficiency: Optimized for performance with a debounce mechanism.
Example in Action:
Hereโs the complete setup in action:
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<meta name="viewport" content="width=device-width, initial-scale=1.0">
<title>Responsive Classes with jQuery</title>
<script src="https://code.jquery.com/jquery-3.6.0.min.js"></script>
<style>
.align-items-center {
align-items: center;
display: flex;
}
.justify-content-center {
justify-content: center;
}
.mobilescr {
height: 100px;
background-color: lightblue;
}
</style>
</head>
<body>
<div class="mobilescr">Resize the window to see the magic!</div>
<script>
function resize() {
if ($(window).width() < 700) {
$('.mobilescr').addClass('align-items-center justify-content-center');
} else {
$('.mobilescr').removeClass('align-items-center justify-content-center');
}
}
$(document).ready(function() {
resize();
});
$(window).on('resize', function() {
resize();
});
</script>
</body>
</html>
Comments
Grabber Pro
Original price was: $59.$39Current price is: $39.Custom WooCommerce Checkbox Ultimate
Original price was: $39.$19Current price is: $19.Android App for Your Website
Original price was: $49.$35Current price is: $35.Abnomize Pro
Original price was: $30.$24Current price is: $24.Medical Portfolio Pro
Original price was: $31.$24Current price is: $24.
Latest Posts
- How to Create a PHP Remote File Downloader with Live Progress Bar
- How to Connect AWS CloudFront URL with a Cloudflare Subdomain
- Android Developer Interview Questions Categorized by Topic
- When Data Must be Sanitized, Escaped, and Validated in WordPress
- Alternative to WordPress for High Traffic News Websites: Node.js & Other Scalable Solutions
Leave a Reply